Connecting Python to WordPress
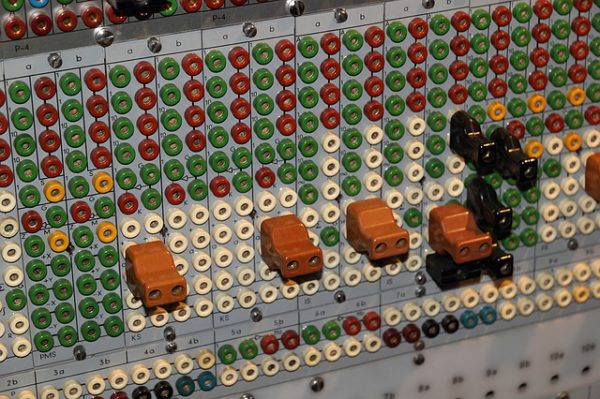
As a part-time engineer, programmer, quant etc, I occasionally have a good code snippet that deserves to be shared. This one is quite simple but powerful. It has already improved my workflow for updating content on this blog. The python package is called wordpress_xml-rpc. My snippet function for uploading content and images (usually jpeg chart images) to WordPress is below.
It is designed to create either “Standard” or “Image” format posts. I initially used “Image” format posts. However, I found that the featured image/thumbail did not show up in the post while reviewing it on Pocket and Feedly . The other images did. I changed back to “Standard” format posts (as you can see below; Image has been commented out). Either way, the featured image specified in FeatImg is still used as the thumbnail for the post.
FeatImg is a string specifying the local file location of the image to be uploaded to WordPress and used as the thumbnail. OthrImgs are complete local filenames to other images that will go into the body of the post, and referenced through html image tags in the text of Body. FeatImg is a string filename argument, OthrImg is a list argument of filename strings. Title, Slug, Body, and Excerpt are all string arguments. Tags and Ctgry are both list arguments of strings.
I used this snippet to produce my last post about Investors Intelligence data here.
from wordpress_xmlrpc import Client, WordPressPost from wordpress_xmlrpc.methods.posts import NewPost from wordpress_xmlrpc.compat import xmlrpc_client from wordpress_xmlrpc.methods import media, posts import os def post_to_wordpress(FeatImg,OthrImg,Title,Slug,Body,Tags,Ctgry,Excerpt,Publish=False): # Connect to wordpress wp = Client('http://www.yourwebsite.here', 'user','password') # Move feature figure to website imagename = os.path.split(FeatImg)[1] imagetype = imagename.split('.')[1] data = {'name': imagename,'type': 'image/'+imagetype} with open(FeatImg, 'rb') as img: data['bits'] = xmlrpc_client.Binary(img.read()) response = wp.call(media.UploadFile(data)) attachment_id = response['id'] # Move secondary figures to website for FileName in OthrImg: imagename = os.path.split(FileName)[1] imagetype = imagename.split('.')[1] data = {'name': imagename,'type': 'image/'+imagetype} with open(FileName, 'rb') as img: data['bits'] = xmlrpc_client.Binary(img.read()) response = wp.call(media.UploadFile(data)) # Create the post post = WordPressPost() post.title = Title post.slug = Slug post.content = Body post.terms_names = {'post_tag': Tags,'category': Ctgry } post.thumbnail = attachment_id post.post_format = 'Standard' # Image post.excerpt = Excerpt post.comment_status = 'open' # Publish if Publish: post.post_status = 'publish' post.id = wp.call(posts.NewPost(post))
Breaking Away
There isn’t any error checking here – I will leave that to you. Coding up semi-automated posts in python might be a little more work initially, but I plan to post charts and insights more frequently so this will save a lot of work down the road. By setting Publish default to False, I am able to go to my WordPress dashboard and give the post a final review and tweak before publishing manually. I don’t plan on making my posts completely automated! Yet, blogging can be time-consuming and figuring this out is another step toward my blogging goal.